· Zen HuiFer · Learn · 需要7 分钟阅读
Top 10 Core Libraries Rust Developers Must Know
Discover Serde for serialization, Rayon for parallel computing, Tokio and Actix-web for asynchronous programming, reqwest as an HTTP client, Diesel for ORM, clap for command-line arguments, Log for flexible logging, Regex for powerful pattern matching, and rand for generating random numbers. These libraries are essential for enhancing development efficiency and code quality in Rust projects.
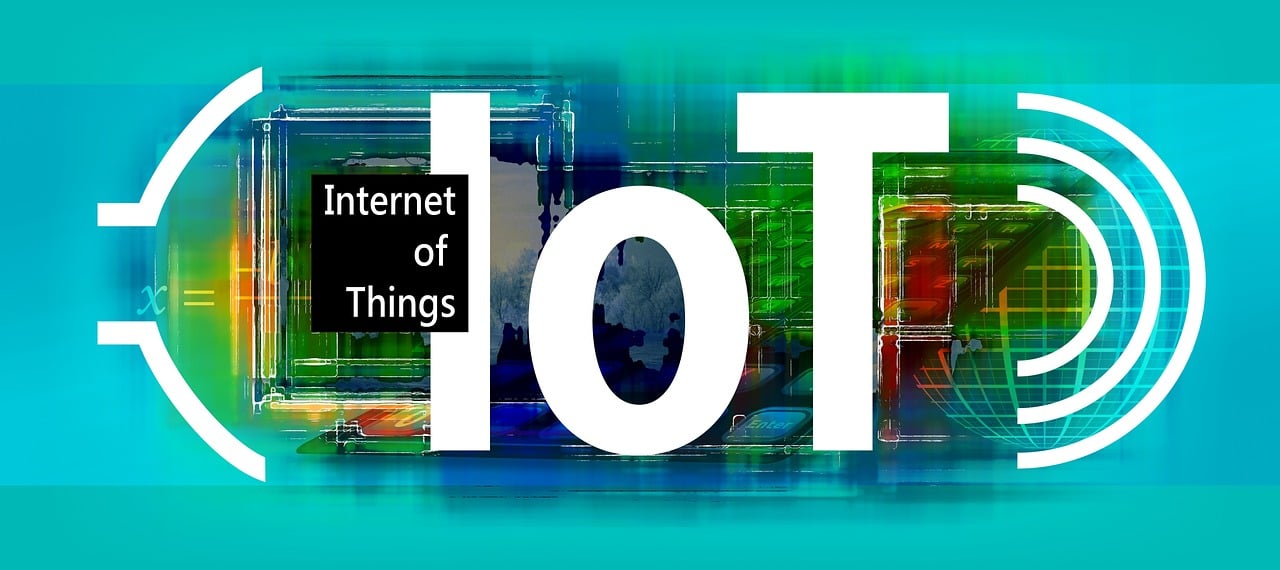
Top 10 Core Libraries Rust Developers Must Know
Rust language has rapidly emerged in the field of software development due to its outstanding performance, security, and concurrent processing capabilities. With the flourishing development of the Rust ecosystem, a large number of libraries known as “crates” have emerged, providing powerful support for various applications from web development to machine learning. A deep understanding and proficient use of these libraries can significantly improve the development efficiency and code quality of Rust projects. This article will delve into ten Rust core libraries, which are tools that every Rust developer should be proficient in.
1. Serde: Easily master serialization and deserialization
In the programming world, serialization is a fundamental and common task that converts data structures into a format that is easy to store or transmit. Serde is the preferred library for serialization and deserialization in the Rust ecosystem. It supports multiple data formats such as JSON, YAML, XML, etc., providing great flexibility for various application scenarios.
Serde is known for its efficiency and low overhead, generating code at compile time to minimize runtime costs. Its powerful ecosystem supports numerous third-party formats, making it an indispensable tool in Rust data exchange.
The main characteristics of Serde are:
Supports multiple data formats JSON, YAML, XML, etc.
Derived macros Automatically generate serialization/deserialization code.
use serde::{Serialize, Deserialize};#[derive(Serialize, Deserialize, Debug)]
struct Point {
x: i32,
y: i32,
}fn main() {
let point = Point { x: 1, y: 2 }; //Serialize to JSON string
let serialized = serde_json::to_string(&point).unwrap();
println!( Serialization result: {} , serialized); //Deserialize from JSON string
let deserialized: Point = serde_json::from_str(&serialized).unwrap();
println!( Deserialization result: {:?} , deserialized);
}
2. Rayon: Unleashing the Power of Concurrent Programming
The performance improvement of modern hardware increasingly relies on the parallel computing capability of multi-core processors. Rayon provides Rust with a simple and easy-to-use concurrent programming model that can easily convert serial code into parallel code, fully utilizing the performance advantages of multi-core CPUs.
The core of Rayon is the job stealing algorithm, which can automatically allocate computing tasks to idle threads, thereby achieving efficient task scheduling and load balancing.
The main characteristics of Rayon are:
Easy to use : Use
par_iter()
Easy implementation of parallel iteration using other methods.Efficient parallel execution Based on job stealing algorithm, automatically perform task scheduling and load balancing.
use rayon::prelude::*;fn sum_of_squares(input: &[i32]) -> i32 {
input.par_iter().map(|&i| i * i).sum()
}fn main() {
let numbers: Vec<_> = (1..1000).collect();
let sum = sum_of_squares(&numbers);
println!( Sum of squares: {} , sum);
}
3. Tokio: The cornerstone of asynchronous programming
With the rapid development of fields such as web applications and network services, asynchronous programming models are receiving increasing attention. Tokio is a leading framework for asynchronous programming in the Rust ecosystem, providing a complete and efficient asynchronous runtime environment that makes it easy to build high-performance, scalable network applications.
Tokio is based on Rust’s asynchronous syntax of asynchronous/await and provides a rich set of asynchronous APIs covering network programming, file system operations, timers, and more.
The main features of Tokio are:
High performance asynchronous runtime Based on efficient event driven models such as epoll/kqueue/iocp.
Rich asynchronous API Provide asynchronous APIs for network programming, file system operations, timers, and more.
use tokio::io::{AsyncReadExt, AsyncWriteExt};
use tokio::net::TcpListener;#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let listener = TcpListener::bind("127.0.0.1:8080").await?; loop {
let (mut socket, _) = listener.accept().await?; tokio::spawn(async move {
let mut buf = [0; 1024]; loop {
match socket.read(&mut buf).await {
Ok(n) if n == 0 => return,
Ok(n) => {
if socket.write_all(&buf[..n]).await.is_err() {
return;
}
}
Err(_) => return,
}
}
});
}
}
4. Actix web: a powerful tool for building high-performance web applications
Web development is one of the important application areas of Rust, and Actix web is a high-performance web application framework in the Rust ecosystem. It is based on the Actix framework, utilizing asynchronous programming models and powerful routing capabilities to easily build high-performance, scalable web applications and APIs.
Actix web adopts a flexible middleware mechanism that allows for easy expansion of functionality and provides support for common web development components such as database connection pools and template engines.
The main features of Actix web are:
High performance Based on asynchronous programming model, it can handle a large number of concurrent requests.
Flexible routing Supports multiple routing rules, making it easy to define API interfaces.
use actix_web::{get, App, HttpServer, Responder};#[get("/")]
async fn index() -> impl Responder {
"Hello, world!"
}#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.service(index)
})
.bind(("127.0.0.1", 8080))?
.run()
.await
}
5. reqwest: A concise and efficient HTTP client
In modern applications, interacting with web services is essential. Reqwest is a powerful and easy-to-use HTTP client library in the Rust ecosystem that provides a concise API for easily sending HTTP requests and processing responses.
Reqwest supports an asynchronous programming model that efficiently handles concurrent requests and provides serialization and deserialization support for common data formats such as JSON and XML.
The main features of reqwest are:
Simple and easy to use Provide a concise API to facilitate sending HTTP requests.
Asynchronous support Supports asynchronous programming model and can efficiently handle concurrent requests.
use reqwest::{Client, Error};#[tokio::main]
async fn main() -> Result<(), Error> {
let client = Client::new();
let response = client
.get("https://www.rust-lang.org")
.send()
.await?; println!( Status code: {} , response.status());
println!( Response content: {} , response.text().await?); Ok(())
}
6. Diesel: A secure and efficient database ORM library
Database is one of the core components of most applications. Diesel is a secure and efficient Object Relational Mapping (ORM) library in the Rust ecosystem, providing a type safe way to interact with databases.
Diesel supports multiple database backends, including PostgreSQL, MySQL, SQLite, etc., and provides compile time query checking to detect database query errors during the compilation phase.
Diesel’s main features:
Type safety Provide type safe APIs to prevent database query errors.
Query check during compilation Check database query syntax during the compilation phase to detect errors as early as possible.
#[macro_use]
extern crate diesel;use diesel::prelude::*;
use diesel::pg::PgConnection;table! {
posts (id) {
id -> Int4,
title -> Varchar,
body -> Text,
published -> Bool,
}
}#[derive(Insertable)]
#[table_name = "posts"]
struct NewPost<'a> {
title: &'a str,
body: &'a str,
}fn main() {
let database_url = "postgresql://user:password@localhost/blog";
let connection = PgConnection::establish(&database_url)
.expect(&format!("Error connecting to {}", database_url)); let new_post = NewPost {
title: "My First Post",
body: "This is the body of my first post.",
}; diesel::insert_into(posts::table)
.values(&new_post)
.execute(&connection)
.expect("Error saving new post");
}
7. clap: elegantly handle command-line parameters
Command line parameter parsing is a common requirement for developing command-line tools and applications. Clap is a powerful and easy-to-use command-line parameter parsing library in the Rust ecosystem, providing a declarative way to define command-line parameters and automatically generate help information and error prompts.
Clap supports multiple parameter types, including flags, options, positional parameters, etc., and provides parameter validation and sub command support.
The main characteristics of clap are:
Declarative syntax Using macros to define command-line parameters is concise and easy to understand.
Automatically generate help information Automatically generate help information and error prompts for user convenience.
use clap::{Arg, Command};fn main() {
let matches = Command::new("My RUST Program")
.version("1.0")
.author("Your Name <your.email@example.com>")
.about("Does awesome things")
.arg(
Arg::new("config")
.short('c')
.long("config")
.value_name("FILE")
.help("Sets a custom config file")
.takes_value(true),
)
.arg(
Arg::new("debug")
.short('d')
.long("debug")
.help("Print debug information verbosely"),
)
.get_matches(); //Get parameter values
if let Some(config_path) = matches.value_of("config") {
println!( Configuration file path: {} , config_path);
} if matches.is_present("debug") {
println!( Debugging mode enabled );
}
}
8. Log: Flexible logging
Logging is an indispensable part of application development, which can help developers track program execution processes, diagnose problems, and analyze performance. Log is a widely used logging library in the Rust ecosystem, providing a flexible logging mechanism that allows for easy output of log information to consoles, files, or other targets.
Log supports multiple log levels, including debugging, information, warning, error, etc., and provides support for log formatting and filtering.
The main features of log:
Multiple log levels Supports multiple log levels, making it easy to control the level of detail in log output.
Flexible logging objectives You can output log information to a console, file, or other destination.
use log::{info, warn, error};fn main() {
env_logger::init(); info!( Program startup ); let result = some_function(); if let Err(err) = result {
error!( Error occurred: {} , err);
} info!( Program ends );
}fn some_function() -> Result<(), String> {
//Simulate a potentially erroneous operation
if true {
warn!( Some unexpected situations have occurred );
return Err( Operation failed .to_string());
} Ok(())
}
9. Regex: A powerful regular expression engine
Regular expressions are an indispensable tool in text processing, which can be used for pattern matching, string replacement, data extraction, and other aspects. Regex is a powerful regular expression engine in the Rust ecosystem that provides Perl compatible regular expression syntax and efficient regular expression matching and replacement functionality.
Regex supports multiple regular expression syntax and provides support for Unicode.
The main features of regex are:
Perl compatible : Supports regular expression syntax compatible with Perl.
Efficient matching Provide efficient regular expression matching and replacement functionality.
use regex::Regex;fn main() {
let re = Regex::new(r"^\d{4}-\d{2}-\d{2}$").unwrap();
let date = "2023-10-26"; if re.is_match(date) {
println!( {} is a valid date format , date);
} else {
println!( {} is not a valid date format , date);
}
}
10. rand: Generate random numbers
Generating random numbers is a fundamental requirement in game development, simulation, cryptography, and other fields. Rand is a commonly used library in the Rust ecosystem for generating random numbers, providing a range of algorithms that can generate high-quality pseudo-random and true random numbers.
Rand supports multiple random number generators and provides control over the distribution of random numbers.
The main characteristics of rand are:
Multiple random number generators Provide multiple random number generation algorithms to meet different needs.
Random number distribution Support multiple random number distributions, such as uniform distribution, normal distribution, etc.
use rand::Rng;fn main() {
let mut rng = rand::thread_rng(); //Generate a random integer between 0 and 100
let random_number = rng.gen_range(0..=100);
println!( Random number: {} , random_number); //Generate a random Boolean value
let random_bool = rng.gen_bool(0.5);
println!( Random Boolean Value: {} , random_bool);
}
summary
The ten Rust core libraries introduced above are just the tip of the iceberg among the many excellent libraries in the Rust ecosystem. With the continuous development of the Rust language, it is believed that more powerful and easy-to-use libraries will emerge, providing Rust developers with a more convenient and efficient development experience.