· Zen HuiFer · Learn · 需要3 分钟阅读
Rust and JVM are deeply integrated to build high-performance applications
Rust and JVM integration paves the way for high-performance applications, blending Rust's memory safety and concurrency with JVM's cross-platform capabilities. This union facilitates efficient native code compilation and robust garbage collection, addressing real-time challenges and startup delays. Explore advanced integration techniques like JNI, GraalVM, and WebAssembly to harness the full potential of both ecosystems for secure and rapid development.
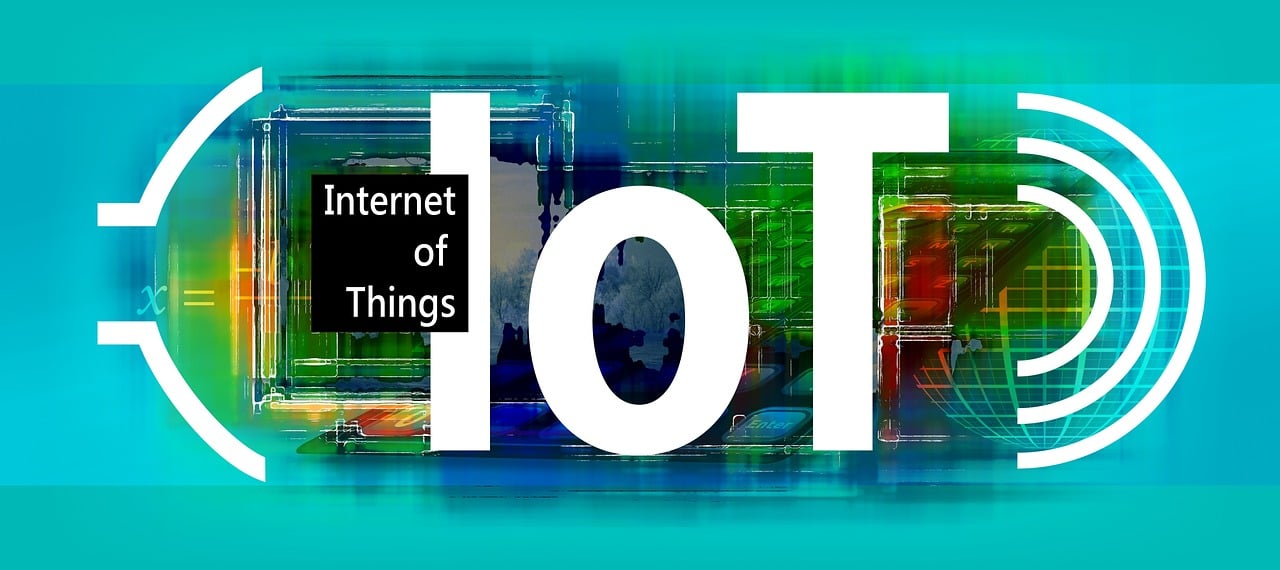
Rust and JVM are deeply integrated to build high-performance applications
In the field of technology, the Java Virtual Machine (JVM) has always dominated with its outstanding performance and cross platform features. At the same time, Rust language has gradually emerged with its advantages of memory safety and concurrency. Combining the two, complementing each other’s strengths and weaknesses, has become a new direction for developers to pursue ultimate performance and security.
Advantages and limitations of JVM
The most notable feature of JVM is its adaptive optimization capability. It can dynamically compile bytecode into optimal local code based on the load situation during program execution, thereby significantly improving program execution efficiency. In addition, the garbage collection mechanism (GC) of JVM greatly reduces the memory management burden of developers and avoids problems such as memory leaks.
However, JVM is not perfect either. Although GC is convenient, its operating mechanism is complex and requires a certain amount of system resources. The triggering timing and execution time of GC are difficult to predict, which is a challenge for applications with high real-time requirements. In addition, the JVM itself is relatively large and takes a long time to start, making it somewhat cumbersome for some lightweight applications.
The complementary advantages of Rust and JVM
The Rust language is known for its memory safety, concurrency, and high performance. Compared to C/C++, Rust’s compiler is able to capture memory related errors at the compilation stage, avoiding the risk of runtime crashes. Meanwhile, Rust’s ownership system and borrowing check mechanism ensure the memory safety of the program in multi-threaded environments.
Combining Rust with JVM can fully leverage the advantages of both to build high-performance and secure applications. For example, you can use Rust to write core modules that are demanding on performance, compile them into dynamic link libraries, and then call them in the JVM. This can not only leverage Rust’s high performance, but also enjoy the convenience brought by the JVM ecosystem.
Integration of Rust and Java Based on JNI
JNI (Java Native Interface) is a mechanism provided by Java for calling local code. Through JNI, Java code can interact with code written in languages such as C/C++and Rust.
Here is a simple example demonstrating how to use JNI to implement Java calling Rust code:
1. Define Java interfaces
public class RustIntegration {
public native int doubleValue(int input); static {
System.loadLibrary("rust_lib"); //Load Rust dynamic library
}
}
2. Generate C-header files
usejavac
Compile Java code with commands and usejavah
Generate a C header file using the command.
javac RustIntegration.java
javah RustIntegration
3. Implement Rust functions
In Rust code, using#[no_mangle]
Attribute tagging functions to prevent function names from being confused.
#[no_mangle]
pub extern "system" fn Java_RustIntegration_doubleValue(env: JNIEnv, _obj: JObject, input: jint) -> jint {
input * 2
}
4. Compile Rust code
usecargo build --target=x86_64-unknown-linux-gnu --release
The command compiles Rust code into a dynamic link library.
5. Run Java code
public class Main {
public static void main(String[] args) {
RustIntegration rustIntegration = new RustIntegration();
int result = rustIntegration.doubleValue(10);
System.out.println("Result: " + result); //Output Result: 20
}
}
Exploring the Integration of Rust and JVM in Depth
In addition to JNI, there are other ways to integrate Rust with JVM, such as:
**GraalVM:**GraalVM is a high-performance multilingual virtual machine that supports running LLVM bytecode. Since Rust can be compiled into LLVM bytecode, GraalVM can be used to run Rust code and interact with Java code.
**WebAssembly:**WebAssembly is a web-based binary instruction format that can run in browsers and other environments. Rust code can be compiled into WebAssembly modules and then used in the JVM.
summary
The combination of Rust and JVM provides new ideas for building high-performance and secure applications. Through JNI or other integration methods, the advantages of both can be fully utilized to meet the needs of different scenarios. With the continuous development of the Rust language and JVM ecosystem, it is believed that the integration between the two will become even closer, bringing more surprises to developers.