· Zen HuiFer · Learn · 需要3 分钟阅读
Lightweight Rust Asynchronous Runtime
Smol is a lightweight and fast asynchronous runtime for Rust, perfect for enhancing I/O operations and network communication efficiency. It supports native async/await, requires minimal dependencies, and is easy to use with its clear API. Ideal for both beginners and experienced Rust developers seeking high-performance async solutions. Learn how to implement Smol with detailed examples.
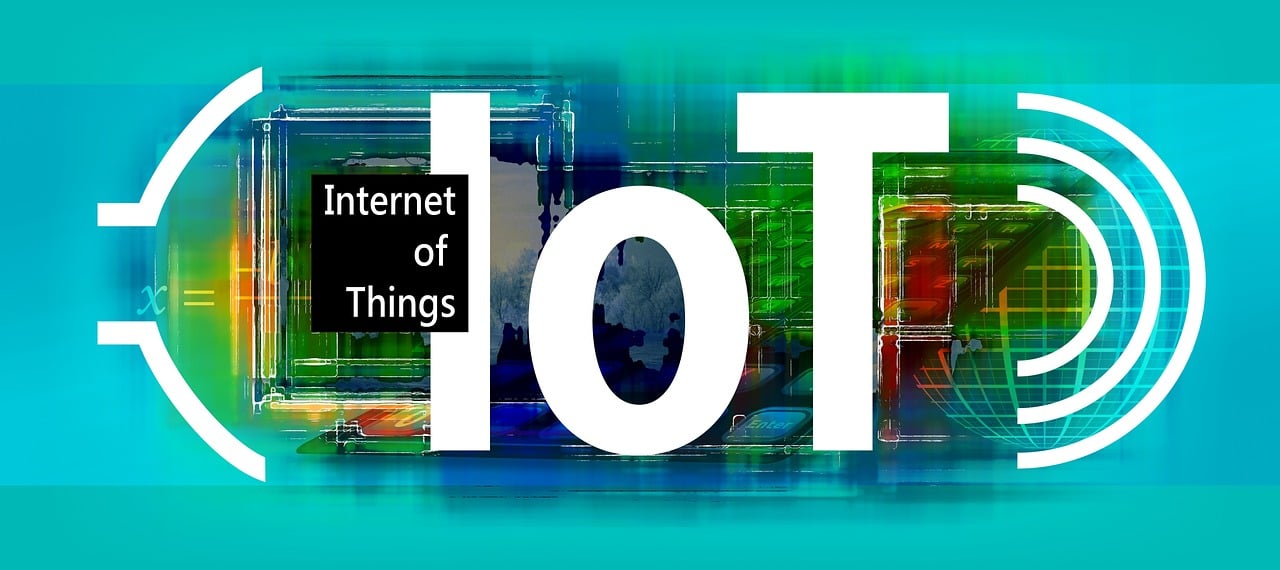
Lightweight Rust Asynchronous Runtime
In the world of Rust programming language, asynchronous programming is an important means to improve program efficiency and performance. In modern software development, with the increasing demand for concurrent programming, asynchronous runtime processing of tasks such as I/O operations and network communication has become particularly important. Smol is a lightweight and fast asynchronous runtime in the Rust language. It maintains a concise and clear API while having rich features, which is deeply loved by many developers. Its efficient scheduling capability enables the runtime to support native asynchronous/await and run efficiently. Next, we will delve into Smol and provide detailed examples to help developers better understand and apply it to practical projects.
Smol’s role and characteristics
Smol is an asynchronous runtime specifically designed for Rust. It provides three types of actuators to poll futures:
Thread Local Executor : Used for
Task::local()
The task created.Work theft actuator : Used for
Task::spawn()
The task created.Blocking actuator : Used for
Task::blocking()
、blocking!
、iter()
、reader()
andwriter()
The task created.
Among these three types of actuators, only the blocking actuator will generate threads on its own.
In addition to the actuator, Smol also includes the following key components:
**Reactor * *: Smol uses epoll as its event reactor on Linux/Android, kqueue for MacOS/iOS/BSD, and wepol for Windows. These reactors are waiting for the next I/O event.
**Asynchronous Type * *: Spol can register I/O handlers in the reactor and convert their blocking operations into asynchronous operations, which is particularly useful when performing complex I/O operations.
**Timer Type * *: Spol allows timers to be registered in the reactor and triggered at predetermined time points to perform related asynchronous tasks.
**Run * *: by calling
run()
Function that can run both the executor and polling reactor simultaneously to handle I/O events and timers. At least one thread call is requiredrun()
To notify the future of waiting for I/O and timers.
Smol Practice Example Explanation
To help you better understand the use of Smol, we will demonstrate how to use Smol in your Rust program through a detailed code example.
Create asynchronous TCP connection
In this example, we will use Smol to create an asynchronous TCP connection and send an HTTP request to the server.
use futures::prelude::*;
use smol::Async;
use std::net::TcpStream;fn main() -> std::io::Result<()> {
smol::run(async {
// Async<TcpStream> TCP
let mut stream = Async::<TcpStream>::connect("example.com:80").await?;
//Define HTTP requests
let req = b"GET / HTTP/1.1\r\nHost: example.com\r\nConnection: close\r\n\r\n";
//Send HTTP requests to the server
stream.write_all(req).await?;
//Create an output stream object using smol:: writer
let mut stdout = smol::writer(std::io::stdout());
//Copy server data to standard output
futures::io::copy(&stream, &mut stdout).await?;
Ok(())
})
}
In this example, we first useAsync<TcpStream>::connect()
Create an asynchronous TCP connection and then use.await
Waiting for the connection to complete. After defining the HTTP request, use.write_all(req).await?
Send the request to the server. Next, usesmol::writer()
Create an output stream object and display the server’s data through standard output. The entire asynchronous execution process issmol::run
Completed in the middle.
summary
Smol holds a place in Rust’s asynchronous programming world with its lightweight design, clear API, and outstanding performance. Whether you are a beginner learning Rust or a seasoned developer seeking high-performance asynchronous solutions, Smol can bring value to your project. Through the detailed explanation and examples above, we hope you can master how to use Smol for effective asynchronous programming in Rust projects.