· Zen HuiFer · Learn · 需要2 分钟阅读
The noCopy strategy you should know in Golang
Discover the importance of noCopy in Golang development. Learn how it prevents accidental copying of critical structures like sync primitives. Enhance your Go code safety and efficiency.
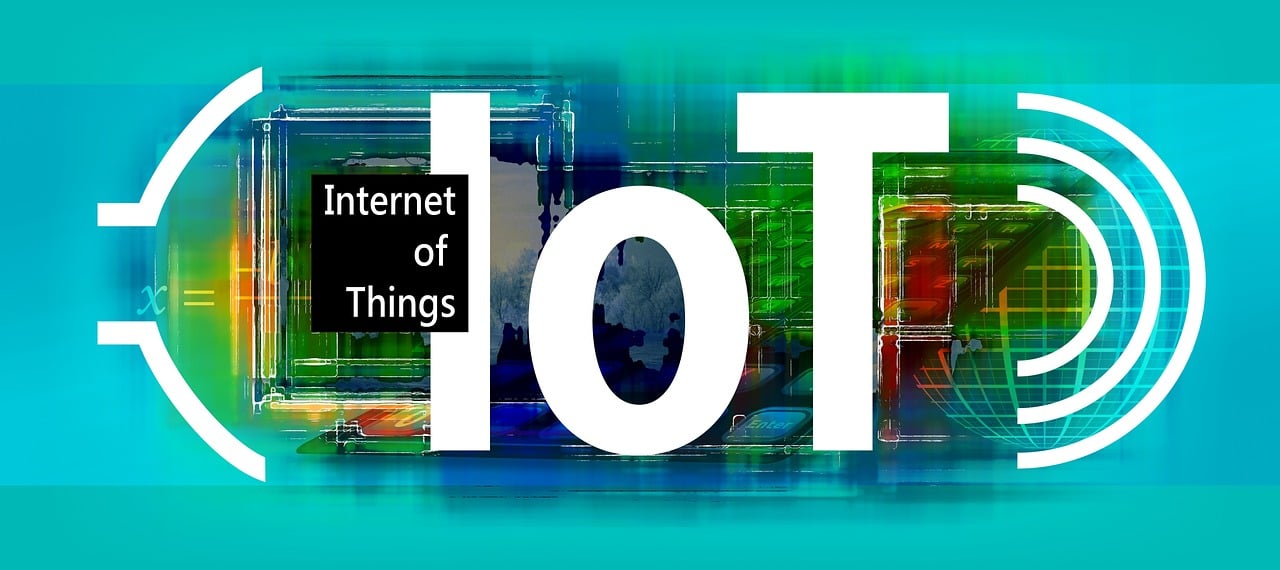
The noCopy strategy you should know in Golang
In Go development, we often encounternoCopy
This structure is accompanied by a common annotation ‘must not be copied after first use’. This article will explore in depthnoCopy
The role of Go Vet and how it can help us avoid potential errors.
sync.noCopy
The role of
sync.noCopy
Structures are usually associated withsync.WaitGroup
Wait for synchronization primitives to appear together, for example:
type WaitGroup struct {
noCopy noCopy state atomic.Uint64 // high 32 bits are counter, low 32 bits are waiter count.
sema uint32
}
noCopy
The structure itself is an empty structure, which implementsLock
andUnlock
Method, both of these methods are null operations. It does not have actual functional attributes, but its existence is of great significance.
Go Vet and ‘Locks Erroneously Passed by Value’
Go Vet is a code checking tool that comes with the Go language, which can help us discover potential code errors.copylocks
It is a checker in Go Vet that checks if a structure containing a lock is incorrectly passed through a value in the code.
For example:
func func1(wg sync.WaitGroup) {
wg.Add(1)
// ...
wg.Done()
}func func2() {
var wg sync.WaitGroup
func1(wg)
wg.Wait() //This will cause errors here
}
stayfunc2
In the middle, we passed it through valueswg
, leading tofunc1
In the middlewg
It has become a new copy. Whenfunc1
In the middlewg
implementDone()
During operation,func2
In the middlewg
Still isAdd(1)
The state ultimately leads towg.Wait()
Cannot function properly.
Go Vet’scopylocks
The checker will detect this error and prompt us with ‘Locks Erroneously Passed by Value’.
noCopy
The mechanism of action
noCopy
Structure through Go Vetcopylocks
The checker cooperates to prevent developers from mistakenly passing structures containing locks through values.
When a structure containsnoCopy
When typing, Go Vet checks whether the structure has been passed through a value. If passed by value, Go Vet will issue a warning to remind developers that the structure should not be copied.
usenoCopy
Other scenes
In addition to synchronization primitives,noCopy
It can also be used in other scenarios where copying needs to be prevented, such as:
Connection Pool: Connection pools usually need to avoid copying connection objects, as copying can cause connection failures.
Resource Management: Resource management objects typically need to avoid replication as it can lead to resource leaks.
Cache: Cache objects usually need to avoid copying, as copying can cause cache invalidation.
summary
noCopy
It is a simple mechanism, but it can effectively prevent developers from mistakenly copying structures containing locks or other important data, thereby avoiding potential errors.
When we seenoCopy
When constructing a structure, one should be aware of its purpose and carefully examine the code to ensure that no structures containing locks or other important data have been copied incorrectly.