· Zen HuiFer · Tutorial · 2 min read
Device Access via COAP
This article details how to use the COAP protocol to access devices on the Go IoT development platform, including access procedures, code examples, and precautions to help developers quickly get started and achieve efficient device access.
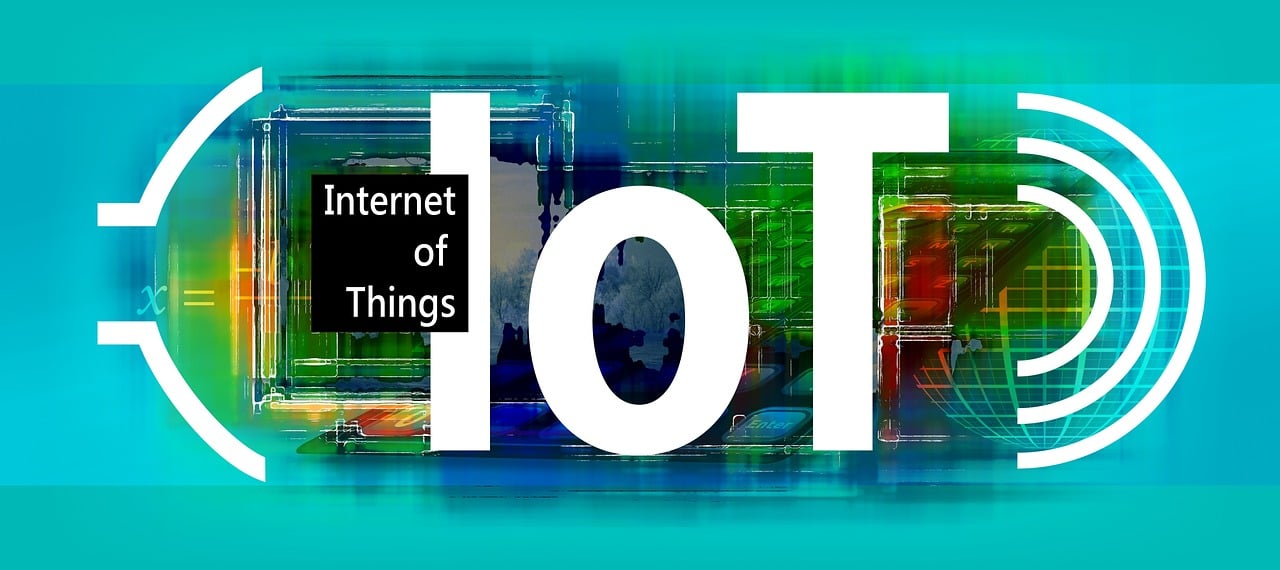
Access Procedure
The demo program is written in Go language, and the COAP server port in a standalone environment is 5683. The complete client program code is as follows.
package main
import (
"encoding/json"
"github.com/dustin/go-coap"
"log"
)
type Auth struct {
Username string `json:"username"`
Password string `json:"password"`
DeviceId string `json:"device_id"`
}
func main() {
c, err := coap.Dial("udp", "localhost:5683")
if err != nil {
log.Fatalf("Error dialing: %v", err)
}
auth(c)
data(c)
}
func data(c*coap.Conn) {
req := coap.Message{
Type: coap.Confirmable,
Code: coap.GET,
MessageID: 12345,
Payload: []byte("test"),
}
path := "/data"
req.SetOption(coap.ETag, "weetag")
req.SetOption(coap.MaxAge, 3)
req.SetPathString(path)
rv, err := c.Send(req)
if err != nil {
log.Fatalf("Error sending request: %v", err)
}
if rv != nil {
log.Printf("Response payload: %s", rv.Payload)
}
}
func auth( c *coap.Conn) {
auth := Auth{
Username: "admin",
Password: "admin",
DeviceId: "1234567890",
}
marshal, _ := json.Marshal(auth)
req := coap.Message{
Type: coap.Confirmable,
Code: coap.GET,
MessageID: 12345,
Payload: marshal,
}
path := "/auth"
req.SetOption(coap.ETag, "weetag")
req.SetOption(coap.MaxAge, 3)
req.SetPathString(path)
rv, err := c.Send(req)
if err != nil {
log.Fatalf("Error sending request: %v", err)
}
if rv != nil {
log.Printf("Response payload: %s", rv.Payload)
}
}
Note: Be sure to use the same connection to complete the data requests for
/auth
and/data
. The server will verify the connection information, and it will not work properly if the two requests are completed with separate connections.
The effect after executing the above program is as follows
2024/08/06 10:16:32 Response payload: 安全认证成功
2024/08/06 10:16:32 Response payload: 数据处理成功